Last Updated | | Ratings | | Unique User Downloads | | Download Rankings |
2017-10-25 (2 years ago)  | | Not yet rated by the users | | Total: 79 | | All time: 9,491 This week: 331 |
|
Description | | Author |
This class can find shopping cart items with most discounts.
It can take an array of items and a list of available coupons to find the those that provide the greatest discount on the whole shopping cart items total.
The class returns a list of possible cart combinations using the different discount coupons that can be applied. Innovation Award
 December 2017
Number 12 |
Many sites implement shopping carts that support applying one or more discount coupons.
Each discount coupon may provide a better discount than others. This class is able to find which coupon of a list is better.
Manuel Lemos |
| |
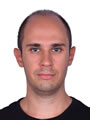 |
|
Innovation award
 Nominee: 5x |
|
Details
ShoppingOptimizer PHP Class
<strong>ShoppingOptimizer </strong> is a <strong>PHP</strong> class that is used to find the most profitable ways to purchase items using cart discount coupons in one or multiple cart parts.
Example script is included in the package. (example.php)<br />
<h2>Sample Outputs</h2>
<ul>
<li>Example with no budget limit (example_output.html) (example_output_sample.png)</li>
<li>Example with budget (example_with_budget_output.html)</li>
</ul>
<h2>Public Methods of Class</h2>
<ul>
<li>getAvailableCartSortOrders()</li>
<li>getCartSortOrders()</li>
<li>getCoupons()</li>
<li>getDiscountedCarts()</li>
<li>removeMaximumBudget()</li>
<li>resetItems()</li>
<li>setCartSortOrders()</li>
<li>setCoupons()</li>
<li>setDefaultCartSortOrders()</li>
<li>setItems()</li>
<li>setMaximumBudget()</li>
<li>setOnlyDistinctSum()</li>
</ul>
<h2>Class Requirements</h2>
<ul>
<li>PHP 5 >= 5.5.0</li>
</ul>
Thanks for checking out this class! If you have improvement idea or bug fix, please feel free to contribute this class.<br />
<br />
<br />
Ovunc Tukenmez
ovunct@live.com
|
example script output |
|
example script with budget output |
|
Open in a separate window
Open in a separate window
|
Applications that use this package |
|
No pages of applications that use this class were specified.
If you know an application of this package, send a message to the author to add a link here.